How to develop Scalable Programs to be a Developer By Gustavo Woltmann
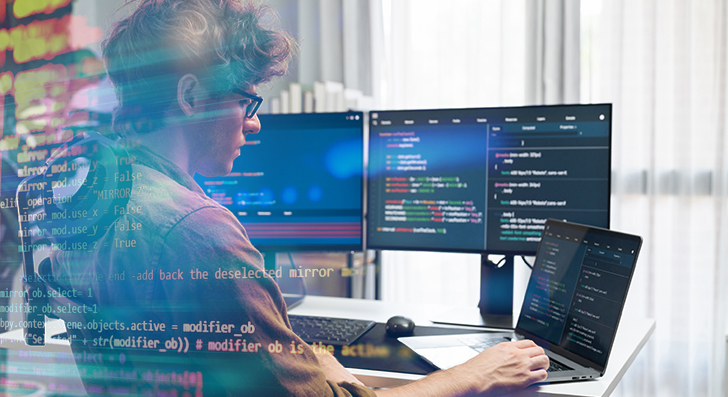
Scalability indicates your application can manage progress—a lot more users, extra knowledge, and even more traffic—without breaking. For a developer, creating with scalability in your mind saves time and stress afterwards. Right here’s a transparent and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on later on—it should be part of your respective strategy from the start. Numerous apps fail whenever they grow rapidly simply because the first design and style can’t tackle the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Start out by designing your architecture to get adaptable. Steer clear of monolithic codebases the place everything is tightly linked. As a substitute, use modular design or microservices. These designs crack your app into more compact, unbiased parts. Each and every module or provider can scale By itself without impacting The full system.
Also, take into consideration your databases from working day a person. Will it require to deal with 1,000,000 people or simply just a hundred? Select the appropriate style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t need them however.
A further vital stage is to prevent hardcoding assumptions. Don’t publish code that only will work less than present-day conditions. Consider what would take place If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that aid scaling, like message queues or event-driven programs. These enable your application cope with additional requests with no receiving overloaded.
If you Construct with scalability in mind, you're not just preparing for success—you might be cutting down upcoming problems. A very well-prepared program is easier to take care of, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the appropriate database is a vital Section of making scalable programs. Not all databases are constructed a similar, and utilizing the Incorrect you can sluggish you down or even bring about failures as your app grows.
Start by comprehending your details. Could it be highly structured, like rows in a very table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They are powerful with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your data is much more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured information and will scale horizontally much more quickly.
Also, think about your browse and publish styles. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Have you been managing a heavy create load? Investigate databases which can deal with large produce throughput, or simply occasion-based mostly details storage methods like Apache Kafka (for short term knowledge streams).
It’s also smart to Believe forward. You may not need to have Highly developed scaling features now, but selecting a database that supports them means you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info dependant upon your entry styles. And generally check database efficiency while you expand.
In a nutshell, the correct database is determined by your app’s construction, speed requirements, And just how you assume it to increase. Get time to pick wisely—it’ll save a lot of hassle afterwards.
Enhance Code and Queries
Rapidly code is key to scalability. As your app grows, each little hold off provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Begin by writing cleanse, basic code. Stay away from repeating logic and take away nearly anything needless. Don’t choose the most elaborate Resolution if a simple a person is effective. Maintain your functions shorter, targeted, and easy to check. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These frequently gradual issues down much more than the code itself. Be certain Each and every question only asks for the data you truly want. Stay clear of Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout massive tables.
For those who recognize the exact same data getting asked for again and again, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat highly-priced operations.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more successful.
Make sure to test with big datasets. Code and queries that get the job done great with 100 records may crash after they have to manage one million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These measures aid your application keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more end users plus much more website traffic. If anything goes as a result of 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. In lieu of just one server undertaking every one of the perform, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data briefly so it may be reused quickly. When people request the same facts once again—like an item website page or even a profile—you don’t really need to fetch it from the databases each time. You can serve it from the cache.
There are 2 typical sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Customer-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And often be certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but highly effective tools. Collectively, they assist your app manage additional users, remain rapid, and Get better from issues. If you intend to develop, you may need both equally.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app increase quickly. That’s where cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you'll need them. You don’t need to acquire hardware or guess potential capability. When targeted traffic boosts, you may insert additional methods with just a couple clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and all the things it ought to run—code, libraries, settings—into one device. This causes it to be simple to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of various containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into companies. You are able to update or scale pieces independently, that's perfect for functionality and dependability.
In short, employing cloud and container resources usually means you'll be able to scale speedy, deploy very easily, and Get better swiftly when complications take place. If you prefer your app to improve with out boundaries, start making use of these applications early. They conserve time, lessen risk, and make it easier to stay focused on making, not correcting.
Check Anything
If you don’t check your software, you received’t know when things go Improper. Checking can help the thing is how your app is executing, place difficulties early, and make better choices as your app grows. It’s a essential A part of creating scalable devices.
Get started by tracking website fundamental metrics like CPU usage, memory, disk Room, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for buyers to load internet pages, how often mistakes occur, and in which they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for important problems. For instance, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair issues fast, normally in advance of buyers even see.
Checking can be beneficial when you make changes. For those who deploy a different characteristic and see a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and information maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, monitoring helps you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about understanding your process and making sure it really works well, even stressed.
Final Ideas
Scalability isn’t only for huge providers. Even tiny applications require a robust Basis. By developing diligently, optimizing correctly, and utilizing the proper applications, you are able to Make apps that expand effortlessly with out breaking under pressure. Start out little, Consider significant, and Develop sensible.